If you are using the Python library requests for web scraping, testing or authentication, you might get stuck on different authentication types from time to time.
A little less common authentication nowadays is HTTP Basic Access Authentication which you often find on older sites such as on-prem local SharePoint sites or similar.
Usually, you will know that the site uses basic authentication if you can authenticate using your credentials as a part of the URL, like for example https://john:123Pass@examplesite.com
.
Just keep in mind that you should never do this for live/production sites as your password will be completely exposed!
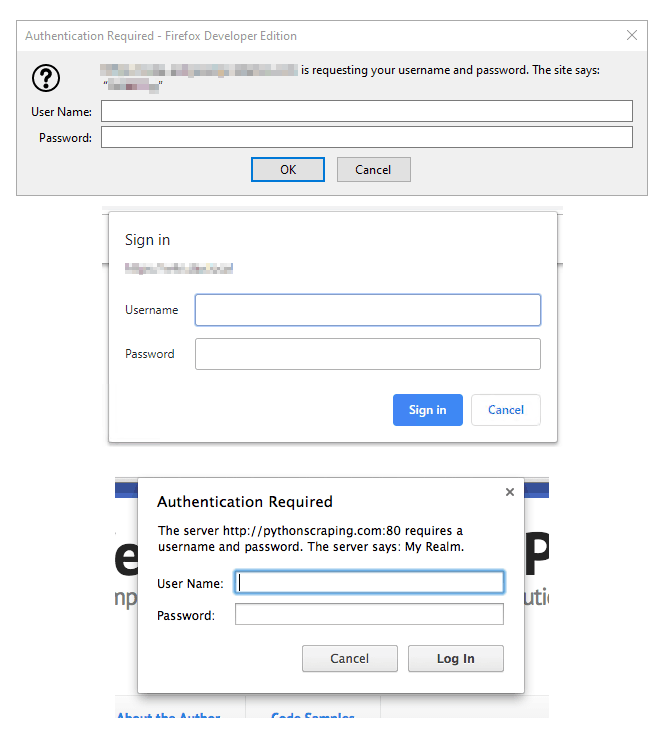
The Requests package in Python contains an auth module specifically designed to handle HTTP authentication called HTTPBasicAuth
.
import requests
from requests.auth import HTTPBasicAuth
auth = HTTPBasicAuth('MyUsername', 'MySuperPassword')
r = requests.get(url = 'https://somewebsite.com', auth=auth, verify=False)
print(r.text)
This will appear as a POST request, but an HTTPBasicAuth object will be passed as an auth argument in the request.
The verify=False
option is only needed if you need to skip SSL certificate verification, handy for sites that do not have SSL certificates perhaps because they are not exposed to the internet.
Sources:
Ryan Mitchell, Web Scraping with Python, 2nd Edition, Page